filter::filter::PadIter doesn't actually iterate
================================================
Bug caught by clippy:
```rust
error: offset calculation on zero-sized value
--> src/filter/filter.rs:98:33
|
98 | let pad = Pad::wrap(self.ptr.offset(self.cur));
| ^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: `#[deny(clippy::zst_offset)]` on by default
= help: for further information visit https://rust-lang.github.io/rust-clippy/master/index.html#zst_offset
```
The problem is that `AVFilterPad` is zero-sized:
```rust
#[repr(C)]
#[derive(Debug, Copy, Clone)]
pub struct AVFilterPad {
_unused: [u8; 0],
}
```
which is in turn due to `AVFilterPad` being an opaque type in `libavfilter/avfilter.h`:
```c
typedef struct AVFilterContext AVFilterContext;
typedef struct AVFilterLink AVFilterLink;
typedef struct AVFilterPad AVFilterPad;
typedef struct AVFilterFormats AVFilterFormats;
```
Doing pointer arithmetic on an opaque (incomplete) type doesn't work.
A short program would expose the bug:
```rust
extern crate ffmpeg_next as ffmpeg;
use ffmpeg::filter;
fn main() {
ffmpeg::init().unwrap();
let filter = filter::find("overlay").unwrap();
for input in filter.inputs().unwrap() {
println!("{} {:?}", input.name().unwrap(), input.medium());
}
}
```
Prints:
```
main Video
main Video
```
Compare this to the C counterpart using the proper APIs:
```c
#include "libavfilter/avfilter.h"
int main()
{
const AVFilter* filter = avfilter_get_by_name("overlay");
for (int i = 0; i < avfilter_pad_count(filter->inputs); i++) {
printf("%s ", avfilter_pad_get_name(filter->inputs, i));
switch (avfilter_pad_get_type(filter->inputs, i)) {
case AVMEDIA_TYPE_VIDEO:
puts("video");
break;
case AVMEDIA_TYPE_AUDIO:
puts("video");
break;
default:
puts("other");
break;
}
}
return 0;
}
```
Prints
```
main video
overlay video
```
We have to tweak our API a bit in order to fix this.
Can't reproduce with googler installed [through choco](https://chocolatey.org/packages/googler).
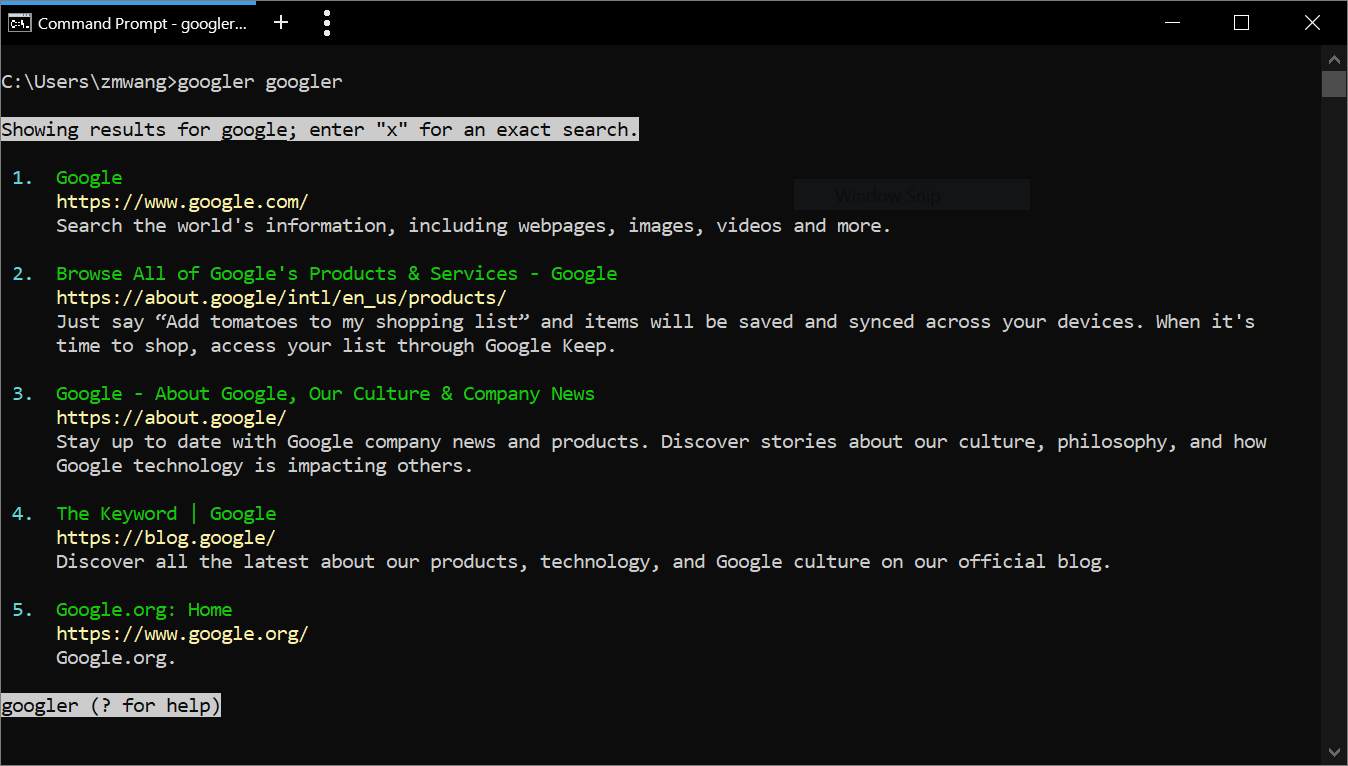
Also, this looks like a generic Python script problem. Start with something simple like placing `argv.py`
```py
import sys
print(sys.argv)
```
on your `PATH` and see if it works.
Since neither of the maintainers here use Windows much, we probably won't be of much help.
This has caused way too much trouble than its worth, my impression is it's been failing half of the time when we used it. I'm also like 99.9% sure no arch user install the package this way -- there's just no reason to not use AUR which should always be on the latest version. Why don't we just remove it.