Fails to read file from archiver.FileSystem when archive is .tar.zst
====================================================================
<!--
This template is for bug reports! (If your issue doesn't fit this template, it's probably a feature request instead.)
To fill out this template, simply replace these comments with your answers.
Please do not skip questions; this will slow down the resolution process.
-->
## What version of the package or command are you using?
v4.0.0-alpha.7
## What are you trying to do?
Extract files from a .tar.zst (zstd) archive with the `archiver.FileSystem` feature.
## What steps did you take?
Minimal reproducible example:
```go
package main
import (
"io"
"log"
"github.com/mholt/archiver/v4"
)
func main() {
archive, err := archiver.FileSystem("test.tar.zst")
if err != nil {
log.Fatal(err)
}
f, err := archive.Open("test")
if err != nil {
log.Fatal(err)
}
if _, err := io.ReadAll(f); err != nil {
log.Fatal(err)
}
}
```
A simple archive `test.tar.zst` with a non-empty `test` file inside it is needed, created through
```console
$ echo 12345678 > test
$ tar -cf test.tar.zst --zstd test
```
## What did you expect to happen, and what actually happened instead?
<!-- Please make it clear what the bug actually is -->
Expected successful read without error. Actually got an error from the `io.ReadAll`:
```
decoder used after Close
```
This is `ErrDecoderClosed` from the zstd module. See https://pkg.go.dev/github.com/klauspost/compress/zstd.
Note that if we use a .tar.gz instead there's no error. The problem is zstd-specific.
Also, `Tar.Extract()` works fine with .tar.zst, so this is specific to the FS implementation.
## How do you think this should be fixed?
<!-- Being specific by linking to lines of code and even suggesting changes will yield fastest resolution -->
I haven't got time to investigate yet.
## Please link to any related issues, pull requests, and/or discussion
<!-- This will help add crucial context to your report -->
## Bonus: What do you use archiver for, and do you find it useful?
<!-- We'd like to know! -->
I use it as a high level interface to archive/tar and compress/gzip, compress/zstd, etc. Saves a lot of boilerplate code.
Noticed another problem with `refactor/mv3`: global `define`s aren't available to option, popup, etc., since compared to the normal `@vite/client`, `mv3client.mjs` does not import `vite/src/client/env.ts` where the `__DEFINES__` are. Not sure what's the best solution, but a quick and dirty way to fix this is to manually bind each define to window in `scripts/client.ts`, e.g.
```ts
declare global {
interface Window {
__APP_VERSION__: string;
}
}
window.__APP_VERSION__ = __APP_VERSION__;
```
Just encountered the same problem. `@types/[email protected]` is causing an additional `Long` type that's incompatible with the builtin `Long` type from `[email protected]`. Had to add `import Long from "long";` to generated `proto.d.ts` to prevent it from picking up the wrong `Long` from `@types/long`.
> I'm having the exact same problems as you @zmwangx. Did you find a solution for these 2 problems?
I already posted a patch for the first problem.
I didn't look into the second one, I just stuck to Windi even though it has some shortcomings.
@tmkx Thanks for the work on manifest v3! I tried the `refactor/mv3` branch ([61b47be](https://github.com/antfu/vitesse-webext/commit/61b47be7f03ab101d4cefda3386db0c9b78ec86a) at the time of writing) and found two problems:
1. `src/background/contentScriptHMR.ts` got removed and the functionality was never restored anywhere, so it seems content scripts are never injected into the page in dev mode. A quick patch of mine:
```diff
diff --git a/src/background/contentScriptHMR.ts b/src/background/contentScriptHMR.ts
new file mode 100644
index 0000000..00687cb
--- /dev/null
+++ b/src/background/contentScriptHMR.ts
@@ -0,0 +1,21 @@
+import browser from 'webextension-polyfill'
+import { isFirefox, isForbiddenUrl } from '~/env'
+
+// Firefox fetch files from cache instead of reloading changes from disk,
+// hmr will not work as Chromium based browser
+browser.webNavigation.onCommitted.addListener(({ tabId, frameId, url }) => {
+ // Filter out non main window events.
+ if (frameId !== 0)
+ return
+
+ if (isForbiddenUrl(url))
+ return
+
+ // inject the latest scripts
+ browser.scripting
+ .executeScript({
+ target: { tabId },
+ files: [`${isFirefox ? '' : '.'}/dist/contentScripts/index.global.js`],
+ })
+ .catch(error => console.error(error))
+})
diff --git a/src/background/index.ts b/src/background/index.ts
index 3b28a58..d2e14e1 100644
--- a/src/background/index.ts
+++ b/src/background/index.ts
@@ -2,6 +2,9 @@ import type { Tabs } from 'webextension-polyfill'
import browser from 'webextension-polyfill'
import { onMessage, sendMessage } from 'webext-bridge'
+if (__DEV__)
+ import('./contentScriptHMR')
+
browser.runtime.onInstalled.addListener((): void => {
// eslint-disable-next-line no-console
console.log('Extension installed')
diff --git a/src/manifest.ts b/src/manifest.ts
index 52b947a..c52ab00 100644
--- a/src/manifest.ts
+++ b/src/manifest.ts
@@ -60,7 +60,7 @@ export async function getManifest() {
// we use a background script to always inject the latest version
// see src/background/contentScriptHMR.ts
delete manifest.content_scripts
- manifest.permissions?.push('webNavigation')
+ manifest.permissions?.push('scripting', 'webNavigation')
}
return manifest
```
Note: this will inject the content script into any page under `host_permissions`; you may need to further limit injection based on `url`.
2. I tried to use this template with Tailwind v3 + PostCSS (the standard setup: https://tailwindcss.com/docs/guides/vite) instead of Windi.css because I'm more familiar with Tailwind and v3 has more features I like. Unfortunately it failed spectacularly with the `MV3Hmr` plugin. Presumably due to some weird interaction between Tailwind's JIT and the `MV3Hmr` plugin's way to detect changes, `writeToDisk` is called in a seemingly infinite loop, and the node process quickly runs out of memory and crashes. I didn't further investigate this.
Hi, I don't use `mpz_imp_raw`, and in any case this lib doesn't read from untrusted input stream or string, so I don't think it's relevant.
I can update gmp if a new version is released, but carrying a patch is way too much trouble. Please kindly remind me again if a new version of gmp is released.
postcss(css-lcurlyexpected) error with Tailwind media query screen() function
=============================================================================
Volar version: v0.29.8
This is similar to #103. I'm using the Tailwind [`screen()` function](https://tailwindcss.com/docs/functions-and-directives#screen) for media queries:
```vue
<style lang="postcss" scoped>
@media screen(sm) {
.CalendarMonth {
width: 24rem;
}
}
</style>
```
This causes an error:
<img src="https://user-images.githubusercontent.com/4149852/146182608-2feb2b02-5771-4f13-9e7d-385fa36c68b1.png" width="394">
Apparently an unsupported syntax. #103 mentioned `<!-- @vue-ignore -->` but support for that has been removed in v0.24.0. https://github.com/johnsoncodehk/volar/discussions/134 says "May support `"css.validate": false` for replacement", but (1) that's not ideal for a single syntax error; (2) AFAICT it's not supported at the moment. Not really sure what can be done to suppress the error.
v6.0.0-alpha.37 is missing dist/vue-tippy.d.ts
==============================================
As stated in the title, the `v6.0.0-alpha.37` npm release is missing `dist/vue-tippy.d.ts` for TypeScript. `v6.0.0-alpha.36` doesn't have this problem.
Supporting that means a parser for the version DSL has to be integrated, and strictly speaking which versions of node are actually available in Homebrew will need to be taken into account too. That's a fair bit of complexity for something the human user can quite easily determine and trivially edit in. Since this tool is about generating the boilerplate parts of a node formula rather than a 100% production-ready formula, I'll decline.
caching-dependencies-to-speed-up-workflows.md: clarify base of a relative path
==============================================================================
<!--
Thank you for contributing to this project! You must fill out the information below before we can review this pull request. By explaining why you're making a change (or linking to a pull request) and what changes you've made, we can triage your pull request to the best possible team for review.
See our [CONTRIBUTING.md](/main/CONTRIBUTING.md) for information how to contribute.
For changes to content in [site policy](https://github.com/github/docs/tree/main/content/github/site-policy), see the [CONTRIBUTING guide in the site-policy repo](https://github.com/github/site-policy/blob/main/CONTRIBUTING.md).
We cannot accept changes to our translated content right now. See the [types-of-contributions.md](/main/contributing/types-of-contributions.md#earth_asia-translations) for more information.
Thanks again!
-->
### Why:
Closes https://github.com/github/docs/issues/12852.
<!--
- If there's an existing issue for your change, please link to it.
- If there's _not_ an existing issue, please open one first to make it more likely that this update will be accepted: https://github.com/github/docs/issues/new/choose. -->
### What's being changed:
<!-- Share artifacts of the changes, be they code snippets, GIFs or screenshots; whatever shares the most context. If you made changes to the `content` directory, a table will populate in a comment below with the staging and live article links -->
### Check off the following:
- [ ] I have reviewed my changes in staging (look for "Automatically generated comment" and click **Modified** to view your latest changes).
- [ ] For content changes, I have completed the [self-review checklist](https://github.com/github/docs/blob/main/contributing/self-review.md#self-review).
### Writer impact (This section is for GitHub staff members only):
- [ ] This pull request impacts the contribution experience
- [ ] I have added the 'writer impact' label
- [ ] I have added a description and/or a video demo of the changes below (e.g. a "before and after video")
<!-- Description of the writer impact here -->
Caching dependencies to speed up workflows: misleading documentation about using relative paths in actions/cache@v2
===================================================================================================================
### Code of Conduct
- [X] I have read and agree to the GitHub Docs project's [Code of Conduct](https://github.com/github/docs/blob/main/CODE_OF_CONDUCT.md)
### What article on docs.github.com is affected?
https://docs.github.com/en/actions/advanced-guides/caching-dependencies-to-speed-up-workflows
### What part(s) of the article would you like to see updated?
In the "Using the cache action" section, under "Input parameters for the cache action":
> `path`: Required The file path on the runner to cache or restore. The path can be an absolute path or relative to the working directory.
The "relative to the working directory" part is misleading. The base of a relative path is in fact the GitHub workspace directory; it is not affected by `run.working_directory` of the step. I found this out in a job where I have a custom `defaults.run.working_directory`.
### Additional information
_No response_
yarn add netlify-cli fails with "JavaScript heap out of memory"
===============================================================
**Describe the bug**
Starting from some time within the past 24 hours, `yarn add netlify-cli@latest` started failing with "JavaScript heap out of memory". This happens on ubuntu 20.04 on GitHub Actions (see my simple repro repo: https://github.com/zmwangx/netlify-cli-ghactions/runs/4479854634), as well as on local macOS dev machine with ample RAM.
`npm install netlify-cli` doesn't seem to suffer from this problem, and RAM usage doesn't balloon.
**To Reproduce**
1. `yarn add netlify-cli`.
**Configuration**
N/A.
**Expected behavior**
`yarn add netlify-cli` succeeds.
**CLI Output**
See this test GitHub Actions run that does nothing other than `yarn add netlify-cli`: https://github.com/zmwangx/netlify-cli-ghactions/runs/4479854634.
<details>
<summary>yarn's error dump</summary>
```
...
<--- Last few GCs --->
[1566:0x571e060] 72710 ms: Scavenge 2024.2 (2078.9) -> 2021.0 (2079.9) MB, 10.4 / 0.0 ms (average mu = 0.368, current mu = 0.345) allocation failure
[1566:0x571e060] 72730 ms: Scavenge 2025.5 (2079.9) -> 2022.8 (2082.9) MB, 7.1 / 0.0 ms (average mu = 0.368, current mu = 0.345) allocation failure
[1566:0x571e060] 72783 ms: Scavenge 2028.4 (2082.9) -> 2025.4 (2100.7) MB, 23.0 / 0.0 ms (average mu = 0.368, current mu = 0.345) allocation failure
<--- JS stacktrace --->
FATAL ERROR: Reached heap limit Allocation failed - JavaScript heap out of memory
1: 0xb02ec0 node::Abort() [node]
2: 0xa181fb node::FatalError(char const*, char const*) [node]
3: 0xced88e v8::Utils::ReportOOMFailure(v8::internal::Isolate*, char const*, bool) [node]
4: 0xcedc07 v8::internal::V8::FatalProcessOutOfMemory(v8::internal::Isolate*, char const*, bool) [node]
5: 0xea5ea5 [node]
6: 0xeb557d v8::internal::Heap::CollectGarbage(v8::internal::AllocationSpace, v8::internal::GarbageCollectionReason, v8::GCCallbackFlags) [node]
7: 0xeb827e v8::internal::Heap::AllocateRawWithRetryOrFailSlowPath(int, v8::internal::AllocationType, v8::internal::AllocationOrigin, v8::internal::AllocationAlignment) [node]
8: 0xe796aa v8::internal::Factory::NewFillerObject(int, bool, v8::internal::AllocationType, v8::internal::AllocationOrigin) [node]
9: 0x11f2e86 v8::internal::Runtime_AllocateInYoungGeneration(int, unsigned long*, v8::internal::Isolate*) [node]
10: 0x15e7879 [node]
/home/runner/work/_temp/d7867e80-8109-416e-9615-2c70737cb77e.sh: line 1: 1566 Aborted (core dumped) yarn global add netlify-cli
```
</details>
**Additional context**
I tried installing older versions of netlify-cli, including even v6.0.0, same issue. But this issue only started happening in my CI builds some time within the last 24 hours, so it's probably some dependency that's causing the problem. I'm not sure how to pin it down though.
Actually upon further investigation, `VNodeChild` only causes an error with `[email protected]`, not newer versions, so I guess `RenderFunction` is okay. Just avoid the problematic version of `vue-tsc`.
Fix vue TypeScript declarations, again
======================================
I'm not sure why `DefineComponent` was changed to `RenderFunction` in #322, but it has a wrong signature (returns `VNodeChild` instead of `VNode`) and is now causing #516 with newer versions of `vue-tsc` (allegedly, I didn't downgrade it to check):
```
error TS2786: '...' cannot be used as a JSX component.
Its return type 'VNodeChild' is not a valid JSX element.
Type 'undefined' is not assignable to type 'Element | null'.
```
as documented in #516. So here we change `RenderFunction` back to `DefineComponent`.
Proxy support is broken
=======================
**Describe the bug**
Proxy support was introduced in #1215, but it is currently broken.
**To Reproduce**
Steps to reproduce the behavior:
1. Somehow make api.netlify.com unavailable, e.g. by being on a closed network where a proxy is required. For reproduction purposes, one can force this by modifying `/etc/hosts` and pointing api.netlify.com to a local container / VM where we spin up a trivial HTTP server that simply stalls on any incoming request.
2. Have a proxy server set up, e.g. mitmproxy or Charles.
3. Run any command through a proxy, e.g. `HTTP_PROXY=http://localhost:8888 netlify login`.
At this point `netlify-cli` would simply stall, and if you monitor the proxy server you'll notice that no request is going through it.
**Configuration**
N/A.
**Expected behavior**
Any network request originating from netlify-cli should be proxied.
**CLI Output**
N/A.
**Additional context**
This happens because `getConfig` happens before the proxy is even configured (with `getAgent`)
https://github.com/netlify/cli/blob/7a55e9871059ac656096735d1c0369b4d473e07e/src/utils/command.js#L46-L57
`getConfig` calls `resolveConfig` which is `@netlify/config`.
https://github.com/netlify/cli/blob/7a55e9871059ac656096735d1c0369b4d473e07e/src/utils/command.js#L4
Looking at `@netlify/config`'s `resolveConfig`: https://github.com/netlify/build/blob/6813d73a21a6c4a6b99576c5b7bc60fae5d8bac4/packages/config/src/main.js#L32-L36 we see that there are API requests made at this stage unless `offline` is true. These requests obviously aren't going through the proxy.
I guess to actually fully support proxies you need to add proxy support to `@netlify/config` as well.
---
Knowing the cause of the issue, here's a risky workaround btw: just set `offline` to true in `src/utils/command.js`:
```diff
- const cachedConfig = await this.getConfig({ cwd, state, token, ...apiUrlOpts })
+ const cachedConfig = await this.getConfig({ cwd, state, token, offline: true, ...apiUrlOpts })
```
One can hot edit this directly into `node_modules/netlify-cli/src/utils/command.js`. (Don't complain to me if anything breaks, obviously.)
---
Related: I believe #2016 is exactly this problem.
Fix vue TypeScript declarations
===============================
The declarations generated by #254 aren't correct, leading to tsc errors as seen in #318.
Btw, somewhat related, it might be a good idea to mention browser support in the documentation? https://csv.js.org/stringify/ only seems to mention `csv-stringify`, `csv-stringify/lib/sync` and `csv-stringify/lib/es5`, none of which works in the browser due to the use of Node's Buffer API. I almost gave up before I noticed the undocumented `browser` module, which does work.
I apologize if I missed something.
Documentation for "Track multiple domains/sites" missing a block of code
========================================================================
The second option suggested on the page currently reads:
> If you want everything in a single overview then you can add the domain to the path, instead of just sending the path:
>
> <script data-goatcounter="https://wasmegg.goatcounter.com/count" async src="//gc.zgo.at/count.js"></script>
I was confused since it doesn't look any different from the standard tracking code, but then I realized the code isn't put in a `<pre>` code block, so a script tag is simply eaten by HTML. Screenshot from devtools:
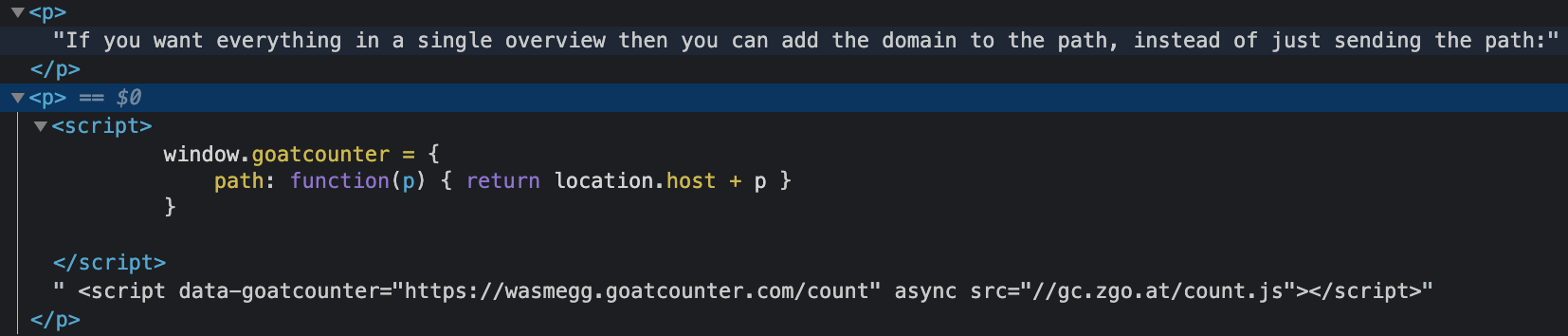
Somewhat related (but probably not the same bug): I noticed another syntax highlighting problem since v0.25.4:
```vue
<template>
<div>
<div>
<div>
<template
v-for="index in 10"
:key="index"
></template>
</div>
</div>
</div>
</template>
```
<img src="https://user-images.githubusercontent.com/4149852/118981803-561aa680-b9ad-11eb-84e3-29dff8bd0bd4.png" width="420">
At a certain point tags become plaintext.
This piece of code actually highlights alright in HTML mode.
Should I open a new issue?
Thanks for looking into the upstream issue! Unfortunately they delegate it to textmate/html.tmbundle which seems to hardly get any attention, and the issue has been outstanding for years: https://github.com/textmate/html.tmbundle/issues/101 I wonder if it's even worth the effort to submit your PR there instead.